Our new Remix portal has been live for over six months now, and we recently received a complaint from a user regarding the date range filter not working in the Safari browser.
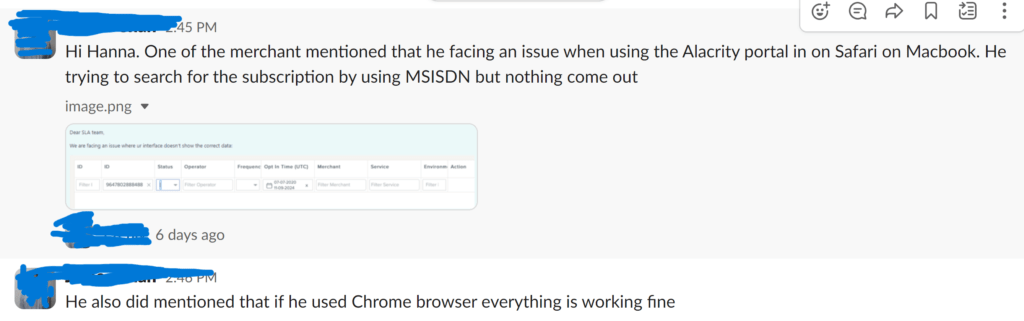
Upon further investigation, we discovered that the issue did not occur in other browsers, which led us to suspect a date compatibility problem specific to Safari.
Thus, the root cause was found in the format of the display date inside the placeholder. In our old portal, the date format was dd/mm/yyyy
, which Safari recognizes as valid. However, in the new portal, we changed the format to dd-mm-yyyy
. It turns out that Safari does not recognize -
(hyphen) as a valid date separator, leading to the issue.
Why Does This Happen?
Safari, unlike some other browsers, has stricter requirements for date format recognition. While dd/mm/yyyy
is widely accepted and recognized by most browsers, dd-mm-yyyy
is not interpreted as a valid date format by Safari. This discrepancy can cause functions that rely on date parsing to fail.
The Solution
To ensure compatibility across all browsers, including Safari, we need to standardize our date format back to dd/mm/yyyy
. Here’s how we can implement this change in our Remix portal:
const fixDates = (dateString) => {
if (typeof dateString === 'string') {
return dateString.replace(/-/g, '/');
}
return dateString;
};
Here how we implement it
const formatToDDMMYYYY = (date) => {
return moment(fixDates(date)).format('DD-MM-YYYY');
};
if (dateInfo && dateInfo.gte && dateInfo.lte) {
const formattedStartDate = formatToDDMMYYYY(dateInfo.gte);
const formattedEndDate = formatToDDMMYYYY(dateInfo.lte);
dateRange = `${formattedStartDate} - ${formattedEndDate}`;
}
By reverting to the dd/mm/yyyy
date format, we can ensure that our date range filter works seamlessly across all browsers, including Safari. This small but crucial change highlights the importance of considering browser-specific quirks when developing web applications.